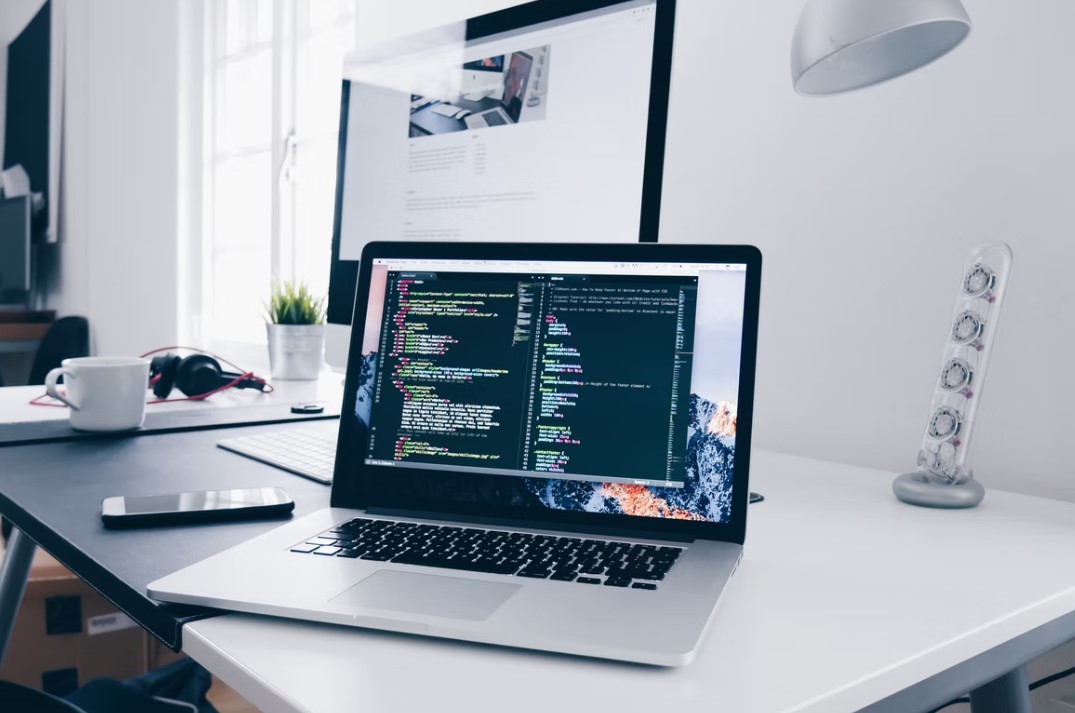
ReactJS has changed the way developers build user interfaces with its modular and reusable approach. Within this framework, React Hooks have simplified state and lifecycle management in functional components, one of the most common needs in many web applications is file uploads.
Why are File Uploads Important in ReactJS?
Modern applications often require users to be able to upload files: images, documents, CSV files, and more. File uploads are crucial in user forms, content management systems, e-commerce platforms, and social networks, among other types of applications.
ReactJS, thanks to its flexible architecture and the introduction of Hooks, offers a simple and efficient way to handle this functionality without relying on classes or components with complex states. With Hooks, we can control file state and upload logic in functional components, resulting in cleaner and more manageable code.

Preparing the environment for file uploads
Before we start with the implementation, let's make sure you have a properly set up ReactJS development environment. If you haven't already, you can create a basic project using the command:
npx create-react-app file-upload
cd file-upload
Once inside the project, let's install Axios, a popular library for making HTTP requests in React. It will allow us to handle file uploads efficiently:
npm install axios
Creating the file upload component
The first step is to create a component that handles file selection and upload. To do so, we'll use the useState Hook to handle the state of the selected file. Here we show you how to implement a basic file upload form.
import React, { useState } from 'react';
import axios from 'axios';
const FileUpload = () => { const [selectedFile, setSelectedFile] = useState(null);
const [isUploading, setIsUploading] = useState(false);
// Handle file selection const handleFileChange = (event) => { setSelectedFile(event.target.files[0]);
};
// Handle file upload const handleUpload = async () => { if (!selectedFile) { alert('Please select a file before uploading.');
return;
} const formData = new FormData();
formData.append('file', selectedFile);
try { setIsUploading(true);
const response = await axios. post('https://api.example.com/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
});
alert('File uploaded successfully');
} catch (error) {
console. error('Error uploading file', error);
alert('There was an error uploading the file.');
} finally {
setIsUploading(false);
}
};
return (
<div>
<h2>File Upload with React and Hooks</h2>
<input type="file" onChange={handleFileChange} />
<button onClick={handleUpload} disabled={isUploading}>
{isUploading ? 'Uploading...' : 'Upload File'}
</button>
</div>
);
};
export default FileUpload;
Code explanation
- State management with useState: We use the useState Hook to manage the selected file (selectedFile) and the upload status (isUploading). This allows us to react to changes in the file and control whether the upload is taking place or not.
- FormData for upload: In the handleUpload method, we use a FormData object to send the file to the server. FormData is a JavaScript API that allows you to send binary data, such as files, in multipart/form-data HTTP requests.
- Axios for HTTP requests: With axios.post, we make the request to the backend, sending the file to the appropriate endpoint. In the example, we have used a test URL, but you can replace it with your API URL.
- Basic validations: Before uploading the file, we verify that the user has selected a file. Additionally, we use a disabled button during upload to avoid multiple requests.
Improving the user experience
The user interface for file uploads can be significantly improved to provide a better experience. Below we will implement some improvements:
- File preview: Show a preview of the selected file, if it is an image.
- Error handling: Implement a messaging system to handle errors during upload.
- Progress bar: Show a progress bar so the user knows how much is left to complete the upload.
Preview Implementation
We can add the functionality to show a preview of the file, especially useful if the file is an image.
const handleFileChange = (event) => {
const file = event.target.files[0];
if (file) {
setSelectedFile(file);
const reader = new FileReader();
reader.onloadend = () => {
setPreviewUrl(reader.result);
};
reader.readAsDataURL(file);
}
};
return (
<div>
<input type="file" onChange={handleFileChange} />
{previewUrl && <img src={previewUrl} alt="Preview" width="200" />}
<button onClick={handleUpload} disabled={isUploading}>
{isUploading ? 'Uploading...' : 'Upload File'}
</button>
</div>
);
Uploading files on the backend
It is essential to make sure that the backend is prepared to receive files via POST requests with multipart/form-data. A simple example in Node.js with Express and Multer would be:
const express = require('express');
const multer = require('multer');
const app = express();
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded.');
}
res.send('File uploaded successfully');
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
This simple example shows how to receive the file on the server using Multer, which is a middleware for handling file uploads in Node.js applications.
File Upload Security
One of the main risks when implementing file uploads is security. Make sure to implement the following measures:
- File Type Validation: Allow only certain file types (such as .png, .jpg images) to prevent users from uploading malicious files.
- File Size Limit: Implement a file size limit to prevent Denial of Service (DoS) attacks.
Uploading files with ReactJS and Hooks is a task that may seem complex at first, but by leveraging the available tools and APIs, we can implement an efficient and flexible solution. With Hooks, it is easier to control the state and lifecycle of components, allowing clean handling of file uploads without having to resort to class components.
If your company needs to integrate file upload functionalities into its applications, at Rootstack we can help you implement custom solutions with the best security and performance practices. Our team of ReactJS experts is ready to take your projects to the next level.
We recommend you on video